WordPress.org Plugin and Theme APIs
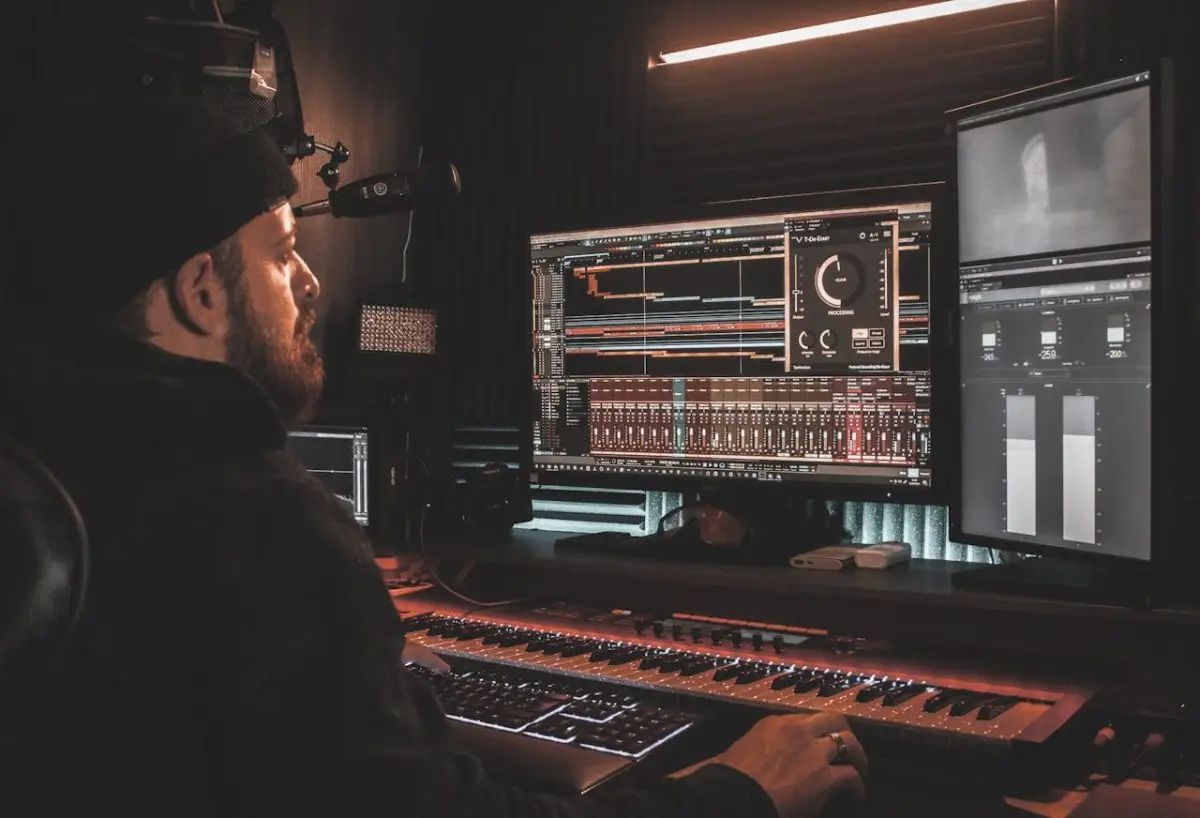
Key Points at a Glance
- About WordPress.org APIs: Users and developers often interact with the WordPress.org Plugin and Theme repositories for tasks like selecting or updating plugins. However, the website lacks features for filtering and sorting by all available fields, hindering tasks such as listing the Most Popular WordPress Themes or the Oldest WordPress Plugins.
- WordPress.org API endpoint: The API endpoint is https://api.wordpress.org/{target}/info/{api_version}, accepting GET requests and returning JSON. A code snippet is provided for querying plugins or themes based on parameters like action, browse, search, tag, or author.
- Querying WordPress.org Plugins and Themes list: Requests can be made to obtain lists of plugins or themes, with parameters for filtering, sorting, and pagination. The provided code snippet serves as a foundation for such queries.
- List of the extra available Theme fields: Additional fields for theme queries are outlined, providing insights into aspects like description, ratings, screenshots, and support URLs.
- Querying WordPress.org about the specific Plugin or Theme: Specific information about a plugin or theme can be retrieved by defining the action as plugin_information or theme_information, respectively. An example response format for both plugins and themes is provided.
Table of Contents
1. About WordPress.org APIs
In everyday WordPress usage, users and developers often interact with the WordPress.org Plugin and Theme repositories either through their browser or their website's dashboard. This suffices for tasks like selecting or updating plugins.
However, while the plugin and theme pages on WordPress.org provide essential information, the website lacks features to filter and sort by all available fields. For instance, you can't easily make a list of the Most Popular WordPress Themes or the Oldest WordPress Plugins, despite WordPress.org having the necessary data.
Another scenario where this limitation becomes apparent is when you need to dynamically display information about a specific theme or plugin on your website. For example, you might want to showcase the number of installations of a plugin live, without relying on manual updates. Fortunately, all of this can be achieved using the official WordPress.org API.
Although there is an official page in the Codex covering the WordPress.org API, the information provided is outdated and incomplete. Recently, while working on the articles mentioned above, we had to delve into the basics of the WordPress.org API.
Now, we're ready to share our knowledge with you to streamline your research process. Let's review the API endpoint and its arguments.
2) WordPress.org API endpoint, arguments, and code example
The API endpoint is https://api.wordpress.org/{target}/info/{api_version}, where the 'target' can be either plugins or themes, and the current API version is 1.2. The endpoint accepts GET requests only and returns JSON with the results.
One effective way to share this knowledge is by providing a practical example. Below, we present a code snippet that we used to obtain the most popular WordPress plugins and themes:
/**
* @return array<string,mixed>
*/
function query_items( string $type, string $action, string $sort, int $page, string $slug = '' ): ?array {
$url = 'plugins' === $type ?
'https://api.wordpress.org/plugins/info/1.2/' :
'https://api.wordpress.org/themes/info/1.2/';
$query_args = [
'action' => $action,
'browse' => $sort,
'per_page' => 100,
'page' => $page,
'fields' => [
'downloaded' => true,
'creation_time' => true,
'tags' => true,
'active_installs' => true,
'last_updated' => true,
],
];
if ( $slug ) {
$query_args['slug'] = $slug;
}
$res = wp_remote_post( $url, [
'method' => 'GET',
'body' => $query_args,
] );
if ( true === is_wp_error( $res ) ) {
echo 'Query error: ' . $res->get_error_message();
return null;
}
$body = wp_remote_retrieve_body( $res );
$body = json_decode( $body, true );
if ( JSON_ERROR_NONE !== json_last_error() ) {
echo 'Json parsing error: ' . json_last_error_msg();
return null;
}
return $body;
}
So, let's go through the available query fields:
- action = query_plugins | query_themes | plugin_information | theme_information
It defines the request type, in this case query. - browse = popular | new | updated | top-rated
It defines the sorting of the results. The popular is the default value, and the order by default is DESC, and we haven't found a way to change it. So if you need the opposite, you've to get the list of pages initially, and then start your research from the latest one. - [search] = x
It allows you to search by any keyword. - tag = x
It allows you to search by tag. - author = x
It allows you to search by author's nickname. - per_page = 100
Limits results per page, by default, is 23. - page = x
Request the specific page from the results. - slug = x
Must be filled for the plugin_information request. - fields = string[]
By default, the WordPress plugins API provides a response that includes all the primary fields, whereas the WordPress themes API includes only the default fields for the query_themes request. For instance, it lacks the published date. If you require specific fields, you must define them here.
Note: The search parameter cannot be used in conjunction with the author or tag parameters. If it is defined, the author and tag will be ignored. However, you can use the other parameters together. For example, you can use browse (sorting) along with the author to get the most popular plugins by the specific author.
2.1) List of the extra available Theme fields
The following fields are not included by default in the query_themes request, but can be added using the fields query argument:
- description
- downloaded
- downloadlink
- last_updated
- creation_time
- parent
- rating
- ratings
- reviews_url
- screenshot_count
- screenshot_url
- screenshots
- sections
- tags
- template
- versions
- theme_url
- extended_author
- photon_screenshots
- active_installs
- requires
- requires_php
- trac_tickets
- is_commercial
- is_community
- external_repository_url
- external_support_url
- upload_date
Alternatively, you can refer to the source code on WordPress.org to obtain it from there.
2. Querying WordPress.org Plugins and Themes list
This scenario allows you to request a list of all plugins or themes, define a search term, and sort the results by several options.
For the plugin, the request URL is: https://api.wordpress.org/plugins/info/1.2/?action=query_plugins&{search_arg}={x}&browse=popular
For the theme, the request URL is: https://api.wordpress.org/themes/info/1.2/?action=query_themes&{search_arg}={x}&browse=popular
You can use the code snippet above as the base. The filter argument can be either search, author, or tag. The sorting type (browse) can be any of the available options.
Now, let's review the response format in detail. Below we provide the response example with the default fields.
2.2) Plugin's query response
$result = [
'info' => [
'page' => 1,
'pages' => 1000,
'results' => 10000,
],
'plugins' => [
[
'name' => 'Plugin name',
'slug' => 'plugin-name',
'version' => 'current plugin version',
'author' => '<a href="http://author-website.com">Author name</a>',
'author_profile' => 'http://author-website.com',
// minimum WordPress version.
'requires' => '5.0',
// tested up to WordPress version.
'tested' => '5.8',
'requires_php' => '7.0',
'requires_plugins' => [],
'rating' => 92,
'ratings' => [
'1' => 10,
'2' => 5,
'3' => 3,
'4' => 2,
'5' => 100,
],
'num_ratings' => 120,
'support_threads' => 10,
'support_threads_resolved' => 5,
'active_installs' => 1000,
'downloaded' => 10000,
'last_updated' => 'Y-m-d H:i:s',
// published date.
'added' => 'Y-m-d',
'homepage' => 'http://plugin-homepage.com',
// that one which shown in the search results.
'short_description' => 'Short description',
'description' => 'Full description',
'download_link' => 'http://downloads.wordpress.org/plugin/plugin-name.zip',
'tags' => [
'some-name' => 'Some name',
],
'donate_link' => '',
'icons' => [
'1x' => 'https://ps.w.org/x',
'svg' => 'https://ps.w.org/x',
]
],
],
];
2.3) Theme's query response
$result = [
'info' => [
'page' => 1,
'pages' => 1000,
'results' => 10000,
],
'themes' => [
[
'name' => 'Theme name',
'slug' => 'theme-name',
'version' => 'current theme version',
'author' => [
'user_nicename' => 'author-name',
'profile' => 'https://profiles.wordpress.org/x',
'avatar' => 'https://secure.gravatar.com/avatar/x',
'display_name' => 'Author name',
'author' => 'Author name',
'author_url' => 'http://author-website.com',
],
'screenshot_url' => 'https://ps.w.org/x/screenshot-1.png',
'rating' => 0,
'num_ratings' => 0,
'homepage' => 'http://theme-homepage.com',
'description' => 'Full description',
'template' => 'parent-theme-slug',
'parent' => [
'slug' => 'parent-theme-slug',
'name' => 'parent theme name',
'homepage' => 'http://wordpress.org/themes/x',
],
// minimum WordPress version.
'requires' => '5.0',
'requires_php' => '7.0',
'is_commercial' => false,
'external_support_url' => false,
'is_community' => false,
'external_repository_url' => '',
]
],
];
As you can see, although the responses for plugins and themes differ, they share common primary fields. The top info section includes pagination information along with the total count of results. The plugins or themes key contains the items along with their respective details.
3. Querying WordPress.org about the specific Plugin or Theme
To query information about a specific theme or plugin, we should utilize the same endpoint but define another action. You can use the code snippet mentioned above, passing plugin_information as the action value and the target slug as the slug argument value.
So, for the plugin, the request URL is: https://api.wordpress.org/plugins/info/1.2/?action=plugin_information&slug={slug}
For the theme, the request URL is: https://api.wordpress.org/themes/info/1.2/?action=theme_information&slug={slug}
Tthe response differs predictably. It excludes the info section and contains only information about the individual plugin or theme. Below, we provide it:
3.1) Information response for the plugin
<?php
$result = array(
"name" => "Plugin Name",
"slug" => "plugin-slug",
"version" => "1.0.0",
"author" => "<a href=\"https://example.com\">Author Name</a>",
"author_profile" => "https://profiles.wordpress.org/author-profile/",
"contributors" => array(
"contributor_username" => array(
"profile" => "https://profiles.wordpress.org/contributor-profile/",
"avatar" => "https://example.com/avatar.png",
"display_name" => "Contributor Name"
)
),
// minimum WordPress version required.
"requires" => "5.5",
// tested up to the WordPress version.
"tested" => "6.5.2",
"requires_php" => "7.4",
"requires_plugins" => array(),
"rating" => 100,
"ratings" => array(
"5" => 27,
"4" => 1,
"3" => 0,
"2" => 0,
"1" => 0
),
"num_ratings" => 28,
"support_url" => "https://wordpress.org/support/plugin/slug/",
"support_threads" => 12,
"support_threads_resolved" => 12,
"active_installs" => 2000,
"last_updated" => "Y-m-d H:i:s",
"added" => "Y-m-d",
"homepage" => "https://example.com/plugin-homepage/",
"sections" => array(
'description' => '<p>Plugin description</p>...',
'installation' => '<p>Installation instructions</p>...',
),
"download_link" => "https://downloads.wordpress.org/plugin-download-link.zip",
"upgrade_notice" => array(),
"screenshots" => array(
"1" => array(
"src" => "https://ps.w.org./screenshot-1.png",
"caption" => "Screenshot 1"
),
),
"tags" => array(
"first" => "First",
"another-one" => "Another one",
),
"versions" => array(
"1.0.0" => "https://downloads.wordpress.org/plugin-version-download-link.zip",
),
"business_model" => false,
"repository_url" => "",
"commercial_support_url" => "",
"donate_link" => "",
"banners" => array(
"low" => "https://example.com/banner-low.png",
"high" => "https://example.com/banner-high.png"
),
"preview_link" => "https://wordpress.org/plugins/slug/?preview=1"
);
2.3) Information response for the theme
<?php
$result = array(
"name" => "Theme Name",
"slug" => "theme-slug",
"version" => "1.0.0",
"preview_url" => "https://example.com/theme-preview/",
"author" => array(
"user_nicename" => "author-username",
"profile" => "https://profiles.wordpress.org/author-profile/",
"avatar" => "https://secure.gravatar.com/avatar/avatar-hash?s=96&d=monsterid&r=g",
"display_name" => "Author Name",
"author" => "Author Name",
"author_url" => "https://example.com/author-info/"
),
"screenshot_url" => "//example.com/theme-screenshot.jpg",
"rating" => 0,
"num_ratings" => 0,
"reviews_url" => "https://example.com/theme-reviews/",
"downloaded" => 0,
"last_updated" => "Y-m-d",
"last_updated_time" => "Y-m-d H:i:s",
"creation_time" => "Y-m-d H:i:s",
"homepage" => "https://example.com/theme-homepage/",
"sections" => array(
"description" => "Some description"
),
"download_link" => "https://example.com/theme-download/",
"tags" => array(
"tag1" => "Tag 1",
"tag2" => "Tag 2"
),
"requires" => "5.0",
"requires_php" => "7.0",
"is_commercial" => false,
"external_support_url" => "https://example.com/theme-support/",
"is_community" => true,
"external_repository_url" => "https://example.com/theme-repository/"
);
Afterwords
That's all for the WordPress.org API exploration. We hope this article clarifies the main points for you, and you're now ready to dive into it. Happy requesting!
Thank you for reading! Subscribe to our monthly newsletter to stay updated on the latest WordPress news and useful tips.
Totally useless
Slightly helpful
Very helpful