ACF Image Field
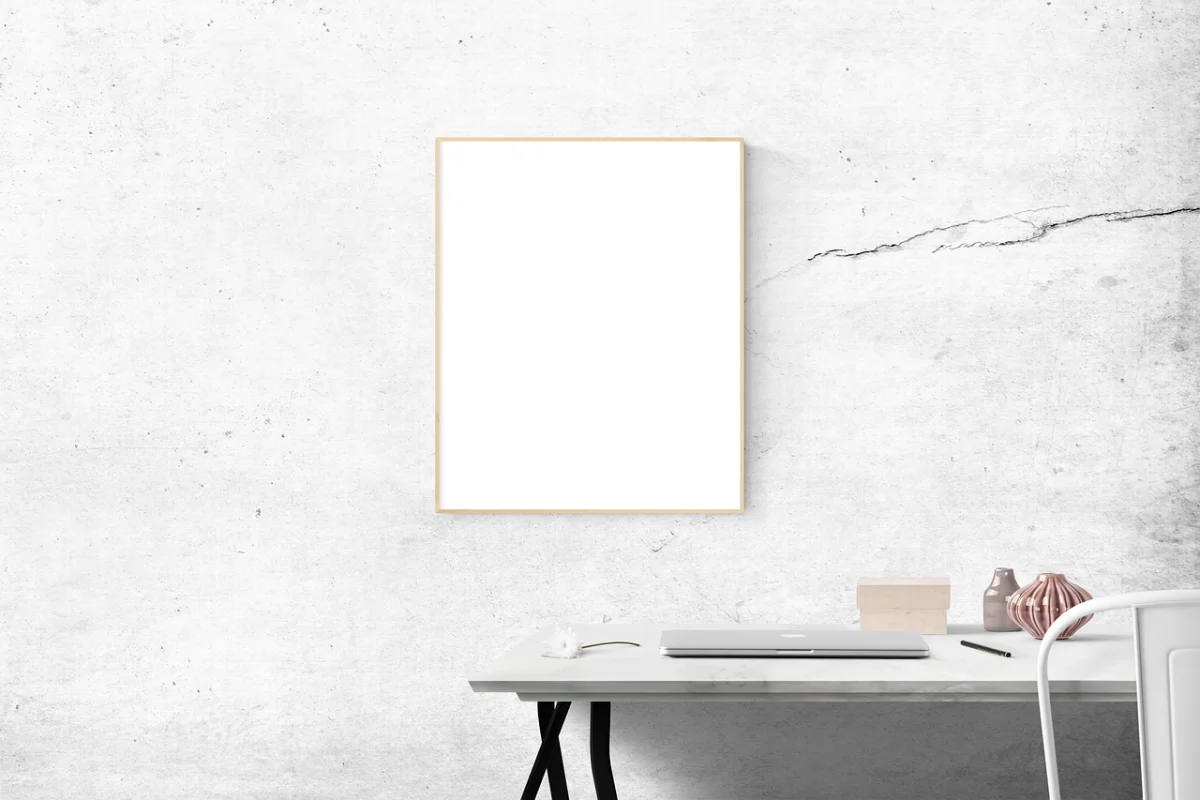
Key Points at a Glance
- ACF Image Field: ACF (Advanced Custom Fields) is a widely-used plugin for managing meta fields and Custom Post Types (CPTs) in WordPress. The Image field is one of its types, enabling selection or upload of images for posts or pages.
- Image Attachments: Unlike WordPress's built-in Featured Image field, ACF Image fields allow attachment of multiple images to posts/pages or even to the options page, providing greater flexibility.
- Extensions and File Types: ACF Image fields support common image file extensions like .jpg, .jpeg, .png, .gif, and .ico. Special hooks are needed for supporting additional file types like .svg.
- Return Formats: ACF Image fields offer three return formats: Image Array, Image URL, and Image ID, each providing different data when retrieving images from the database.
- Behind the Scenes: ACF saves image IDs in the post meta, allowing the same image to be used across multiple pages without duplicating it in the media library.
- Display Methods: Images from ACF fields can be displayed using shortcodes or PHP code, with options to control image size and format.
- Displaying Images with Smart Templates: The Advanced Views framework simplifies the display of ACF fields with smart templates built on the Twig engine, automating template generation and content display.
Table of Contents
About the Image field
Advanced Custom Fields (ACF) is one of the best plugins for managing meta fields and Custom Post Types (CPTs). The Image field is one of the ACF field types, and it allows you to select any image from the WordPress Media Library or upload a new one.
While WordPress Posts and Pages have a built-in Featured Image field, this field only allows a single image for the entire post/page, which can be limiting. By using ACF image fields, you can 'attach' multiple images to your post/page or even to the options page.
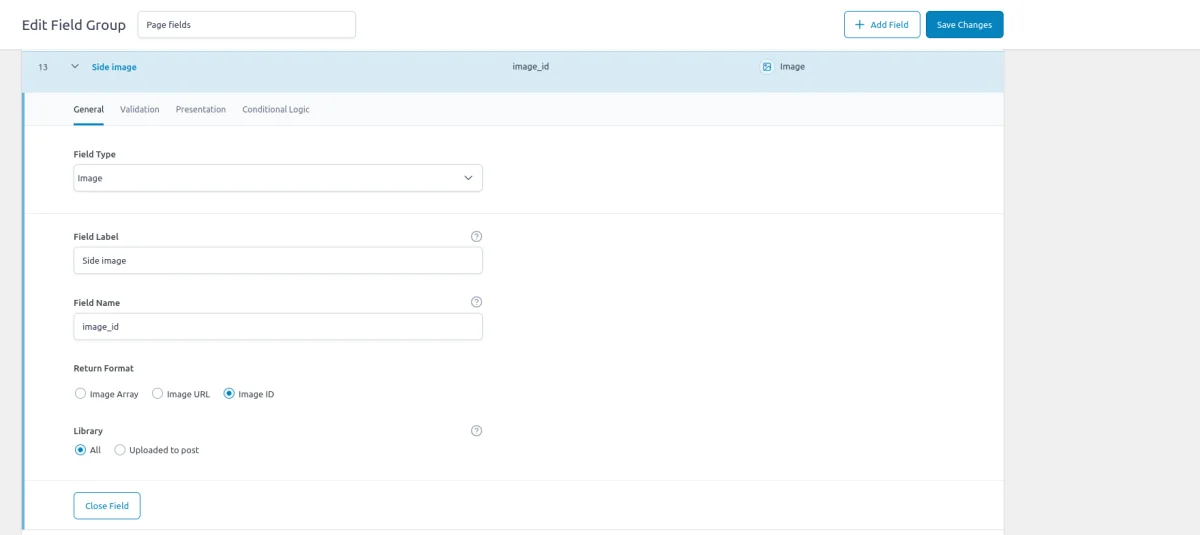
Note: Keep in mind that a single Image field allows you to select only one image. If you wish to add a new image to your page, you'll need to create a new field for each additional image. If you want to select and store multiple images within a single field, consider using the ACF Gallery field, which is specifically designed for this purpose.
Extensions
For images, the allowed extensions are .jpg, .jpeg, .png, .gif, and .ico. You can find a list of allowed extensions for all file types on the related wordpress.org page. If you wish to allow uploading of file types such as .svg, you'll need to utilize special hooks.
Note: It's not possible to upload non-image files to the ACF Image field. For file types like .doc or .pdf, even with the use of hooks, you should consider using the ACF File field, which is designed for this purpose.
If you're interested in learning the hook names to add support for additional image extensions beyond those listed above, we provide a code snippet that enables .svg uploading on your website. You can customize it for your desired extension or use it as is for .svg. The code can be added to your functions.php file. Alternatively, you can use the Safe SVG plugin.
<?php
add_filter('upload_mimes', function ($mimes) {
$mimes['svg'] = 'image/svg+xml';
return $mimes;
});
add_filter('wp_check_filetype_and_ext', function ($data, $file, $filename, $mimes) {
$filetype = wp_check_filetype($filename, $mimes);
return [
'ext' => $filetype['ext'],
'type' => $filetype['type'],
'proper_filename' => $data['proper_filename']
];
}, 10, 4);
Return Formats
The Image field type offers three different Return Formats: Image Array, Image URL, and Image ID. These choices won't affect how the field appears or the options available to site administrators. However, the selected Image Return Format determines the data you receive in the code when you request this image from the WordPress Database using the get_field function.
We recommend selecting the 'Image ID' option as it provides the best performance. It's advisable to avoid the 'Image URL' option unless you have a specific reason for needing it, as it only provides the URL of the image without any additional details, which may not be sufficient for your needs.


Behind the ACF scenes
Here's a behind-the-scenes look at how it works: When you select an image, it saves the ID of the chosen image in the Post Meta of the current Page (or Post/CPT item). This means you can select the same image on many different pages, and it won't create duplicate copies of that image.
When you add a new image using any image field, ACF physically uploads that image to your WordPress installation, placing it in the wp-content/uploads folder. It then creates a new Attachment for this image and saves the ID of the attachment to the current Post/Page's meta.
It's important to note that the ID of an image is saved to the database regardless of the 'Return Format' option. The 'Return Format' option only controls what data you receive in your code. In any case, only the ID of the image (attachment) is stored in the database. That's why we recommend using the 'ID' Return Format, as it aligns with the database format and doesn't require additional processing from the ACF plugin to retrieve it.
Avoid duplicates
Every time you upload a new image, it creates a new attachment in your Media Library. WordPress can't automatically compare the new image to those already uploaded, which means it will create another attachment even if you're uploading the same image again. This is why it's crucial to give images clear names and to first search the Media Library before uploading a new image. Otherwise, you'll accumulate duplicates of the same image, which can lead to increased storage usage on your web hosting account, not to mention potential complications down the line.
Unassigning an image from an image field doesn't remove the image from your WordPress installation. The image file will remain in your Media Library, even when you've removed it from all pages/posts where the image field is used. In fact, there are only a few specific scenarios where you might need to completely remove an image from your WordPress library, but those are beyond the scope of today's article.
Display Image field using Smart templates
Let's get familiar with the Advanced Views framework.
Advanced Views introduces smart templates for effortless content display. It comes with built-in post queries and automated template generation, enabling rapid development while maintaining flexibility.
Let's first clarify what we mean by 'templates' in the context of this plugin: Advanced Views templates are built on the Twig engine. You might be thinking, 'Not bad, but it still requires fetching fields via PHP and writing markup from scratch, not to mention reading Twig documentation'.
Here's where the plugin shines: "Smart templates". This means we don't have to fetch fields or create markup manually from scratch. The plugin provides a solid foundation that covers most use cases. If we require something specific, we can easily customize it. Isn't that nice?
Basics
Now, let's take a basic look at how it works.
The plugin introduces two new Custom Post Types (CPTs): ACF View and ACF Card.
- View for post data and Advanced Custom Fields
We create a View and assign one or more post fields, the plugin then generates a shortcode that we'll use to display the field values to users. We can style the output with the CSS field included in every View. - Card for post selections
We create a Card and assign posts (or CPT items), choose a View (that will be used to display each item) and the plugin generates a shortcode that we'll use to display the set of posts. The list of posts can be assigned manually or dynamically with filters.
The plugin offers us the convenience of working with familiar WordPress CPTs while taking care of querying and automatically generating Twig markup templates. You can find more information about the plugin's benefits here.
To follow along; install the Advanced Views framework on your WordPress site and remember to activate it. You'll also need the ACF (free) plugin installed and active. Then continue to the next steps below.
Step 1. Creating a View
When you activate the Advanced Views framework, you'll notice a new item in your admin menu titled "Advanced Views."
Within the submenu, you'll find several items, but the one you'll need to use is called 'Advanced Views.'
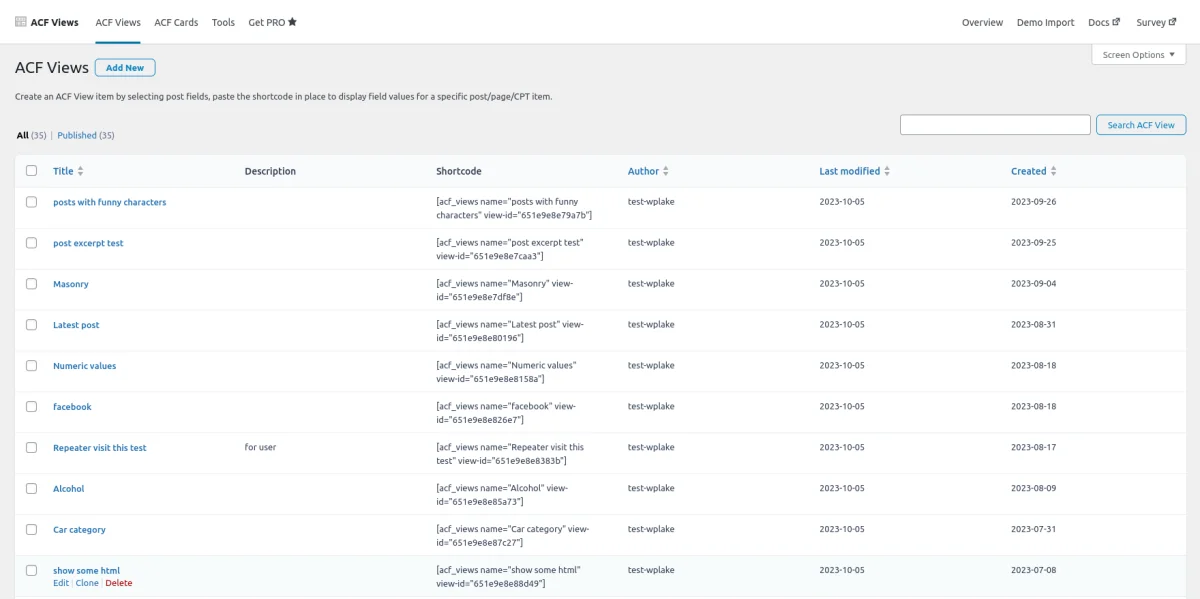
Visit the Advanced Views tab and click the 'Add New' button to create a View.
Provide a name for your View. It can be anything that describes the View, as this name will be displayed in the list of Views, making it easier to identify. For example, we've named our View "Page side image."
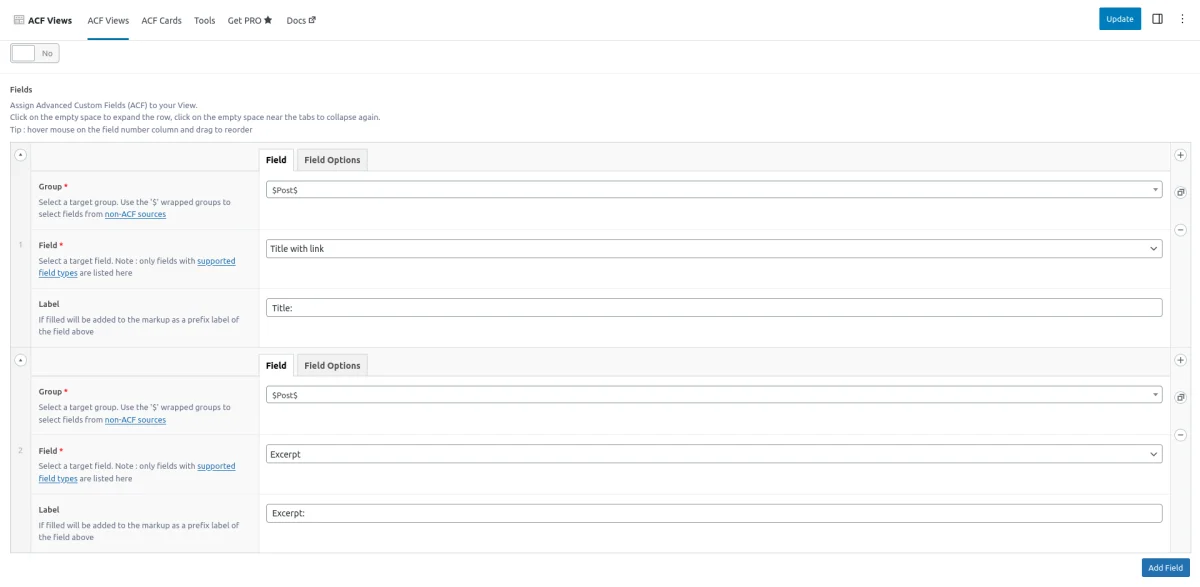
Assigning fields
Now, you need to assign a new field to your View. Click the 'Add Field' button and select your 'Group' from the dropdown. In our case, the group is named "Page fields." Then, select the target field from the list. In our case, the field is named "Side image" (Note: The field type is shown in brackets for easy identification). In this instance, you should see that the type is "image." Continue by choosing an 'Image Size' from the list of available sizes. In our case, we've selected 'Full.'
While every View supports an unlimited number of ACF fields, in our case, we'll use only one.
Click the 'Publish' button to save and publish your View. After your View is published, you'll notice that Shortcodes have been generated in a block on the right side of your View edit screen. Each View has its unique shortcode with a unique ID. Therefore, the shortcode structure is the same for all Views, but the arguments are unique.
[acf_views view-id="xxxx" name="x"]
Click on the 'Copy to clipboard' button for the first shortcode.
Step 2. Paste the shortcode in place
Alright, now everything should be set up to display the image field. Go to the target page with the image field. Ensure that the field has an image attached, and then paste the shortcode wherever you'd like in the page content. To insert the shortcode using the Gutenberg editor, click on the plus button and select the "Shortcode" block from the list. Paste your shortcode into the block and click the 'Update' button to save your post/page.
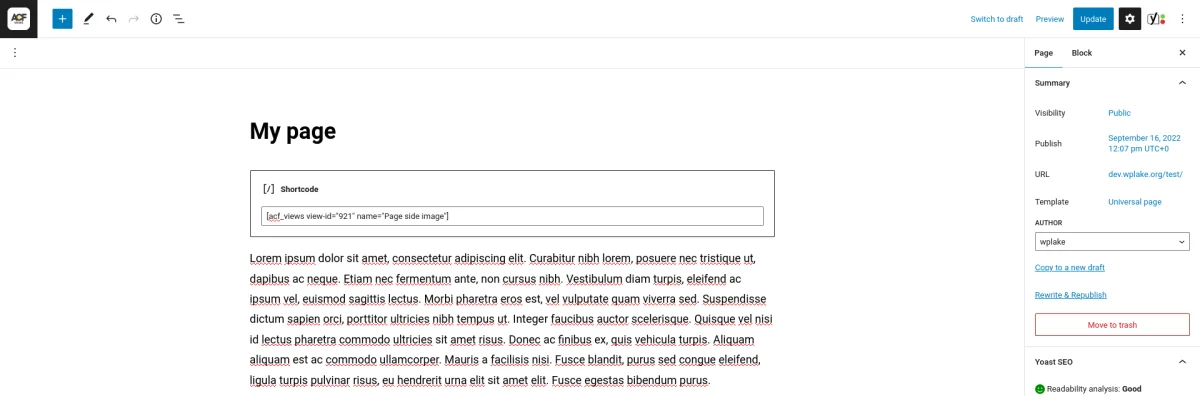
Visit the page to view the result. If you've followed all the steps correctly, you should now see your ACF image displayed within the content.
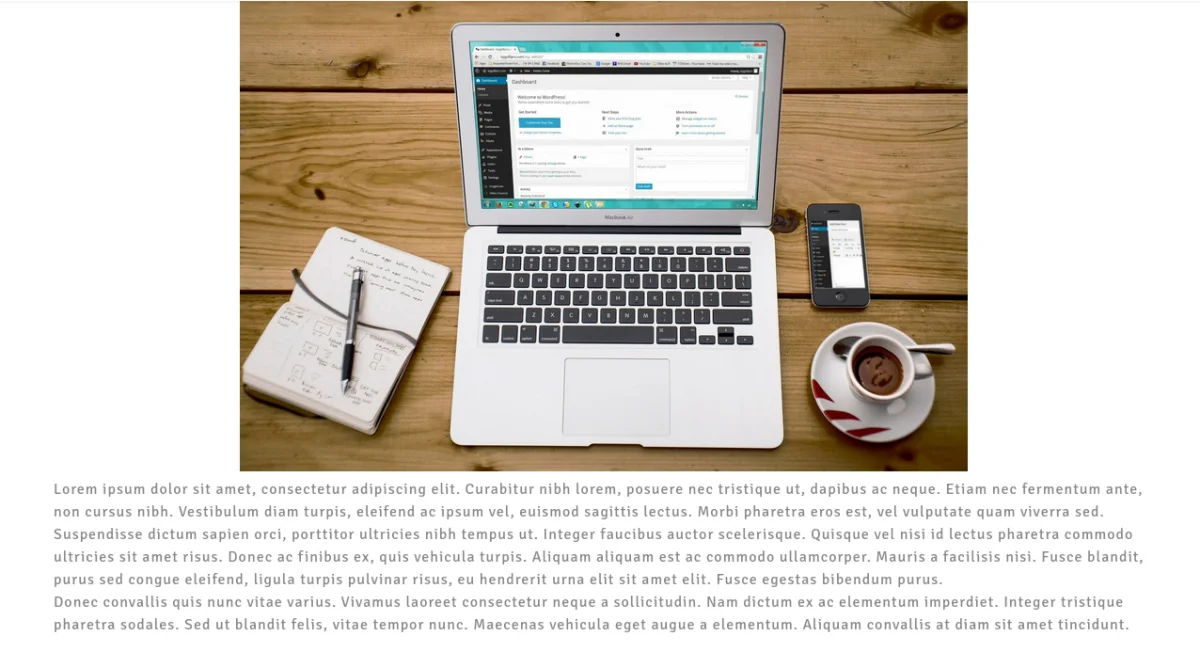
If you can't see your image, go back and edit the page. Ensure that you have attached an image to the ACF image field, as an empty field will not display anything.
Display Image field using PHP code
To display the Image field, we need to convert the selected image into an img tag.
The code will vary depending on the selected Return Type. Below, we have provided examples.
1. PHP code to display the Image field with the "ID" Return Format:
// don't forget to replace 'image' with your field name
$imageID = get_field('image');
// can be one of the built-in sizes ('thumbnail', 'medium', 'large', 'full' or a custom size)
$size = 'full';
if ($imageID) {
// creates the img tag
echo wp_get_attachment_image($imageID, $size);
}
2. PHP code to display the Image field with the "Array" Return Format:
<?php
// don't forget to replace 'image' with your field name
$imageData = get_field('image');
// can be one of the built-in sizes ('thumbnail', 'medium', 'large', 'full' or a custom size)
$size = 'full';
if ($imageData) {
// displays the image. Each %s in the string will be replaced with the related argument
printf("<img src='%s' alt='%s'>",
esc_attr($imageData['size'][$size]), esc_attr($imageData['alt']));
}
3. PHP code to display the Image field with the "URL" Return Format:
// don't forget to replace 'image' with your field name
$imageURL = get_field('image');
if ($imageURL) {
// displays the image. Each %s in the string will be replaced with the related argument
printf("<img src='%s'>",
esc_attr($imageURL));
}
When using the "URL" Return Format, it's important to note that you can't control the image size, and you won't have access to any additional information beyond the URL itself. Therefore, it's recommended to avoid this option and instead choose the "ID" Return Format for more flexibility and control.
For additional information, you can watch the video below and refer to the official ACF article.
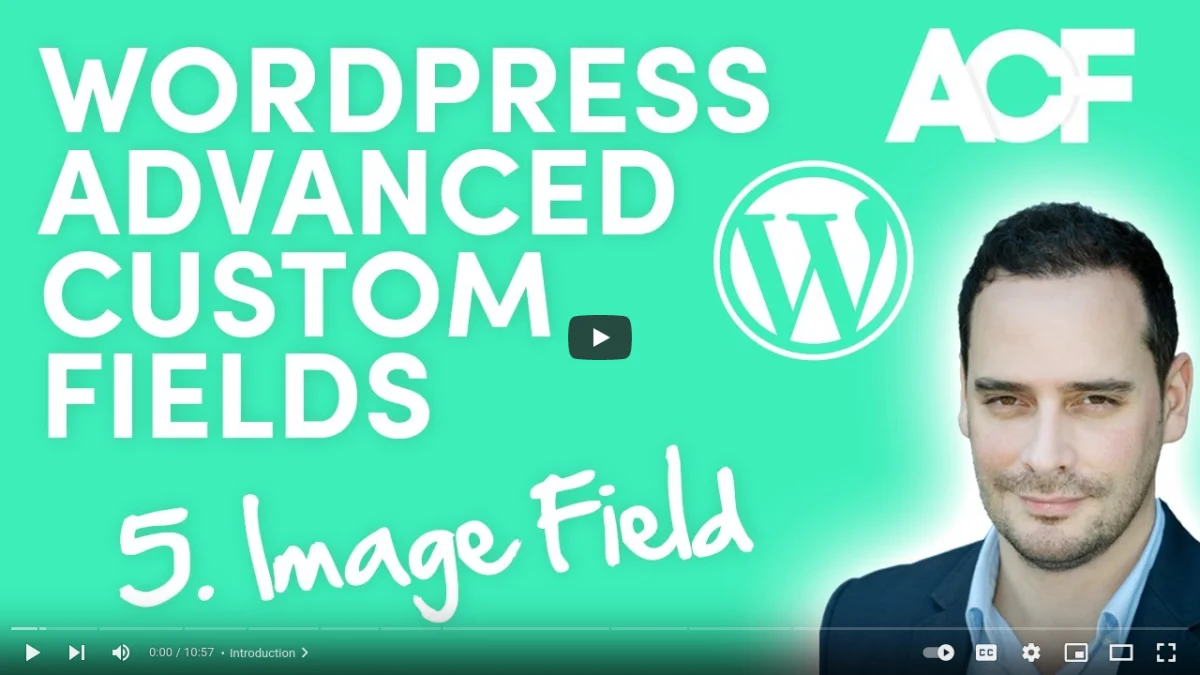
Final thoughts
You have learned how to utilize the ACF Image field, its various options, and two methods to display it. With this knowledge, you can easily incorporate this field into your content.
Remember that you can include more fields of different types within your View. For instance, if you wish to showcase several images with titles on a page. Additionally, you can style field values using the CSS code field, accessible in the View's Advanced Tab. This CSS will only be applied to pages where you've inserted the shortcode.
I trust that this article has been informative. For further information about the Advanced Views framework, please visit the official website. There, you'll find links to the YouTube channel for video tutorials and access to the plugin Documentation, which can assist with any questions you might have.
Thank you for reading! Subscribe to our monthly newsletter to stay updated on the latest WordPress news and useful tips.
Frequently Asked Questions Test Your Knowledge
FAQ mode
/
Learning mode
- What is the ACF Image field, and how does it differ from WordPress's built-in Featured Image?
The ACF Image field is a component of the Advanced Custom Fields plugin, enabling users to attach multiple images to posts or pages, unlike WordPress's Featured Image, which allows only one image per post/page.
- What file types does the ACF Image field support?
The ACF Image field supports common image file extensions such as .jpg, .jpeg, .png, .gif, and .ico. Special hooks are required for additional file types like .svg.
- How does ACF handle image attachments behind the scenes?
ACF saves image IDs in the post meta, allowing the same image to be used across multiple pages without duplicating it in the media library, thus optimizing storage usage.
- What are the available return formats for ACF Image fields, and how do they differ?
The available return formats are Image Array, Image URL, and Image ID. Each format provides different data when retrieving images from the database, offering varying levels of flexibility and control.
- What methods can be used to display images from ACF Image fields?
Images from ACF Image fields can be displayed using shortcodes or PHP code, with options to control image size and format based on user preferences and requirements.
- What are the best practices for managing image uploads with the ACF Image field to avoid duplicates?
It is recommended to give images clear names and search the media library before uploading new images to avoid duplicates, which can lead to increased storage usage and potential complications in the future.
Content links (16)
12.
twig.symfony.com